Today is a special day for those who just are starting out in the JavaScript world. Why? Because it’s well-known that the only way to be master in our craft is through practice. So we are going to dirty our hands and start to improve our DOM manipulation skills. how? We are going to build out a simple arcade game just with Vanilla JavaScript. Great!
Despite this post is for beginners and newbies that doesn’t mean that the more experienced developers are restricted to it though.😎
What we will cover
- The basic of CSS and JavaScript
- The basic of Flexbox, the CSS3 web layout model
- Dynamically manipulate the DOM using JavaScript
- A step-a-step walkthrough
The Challenge
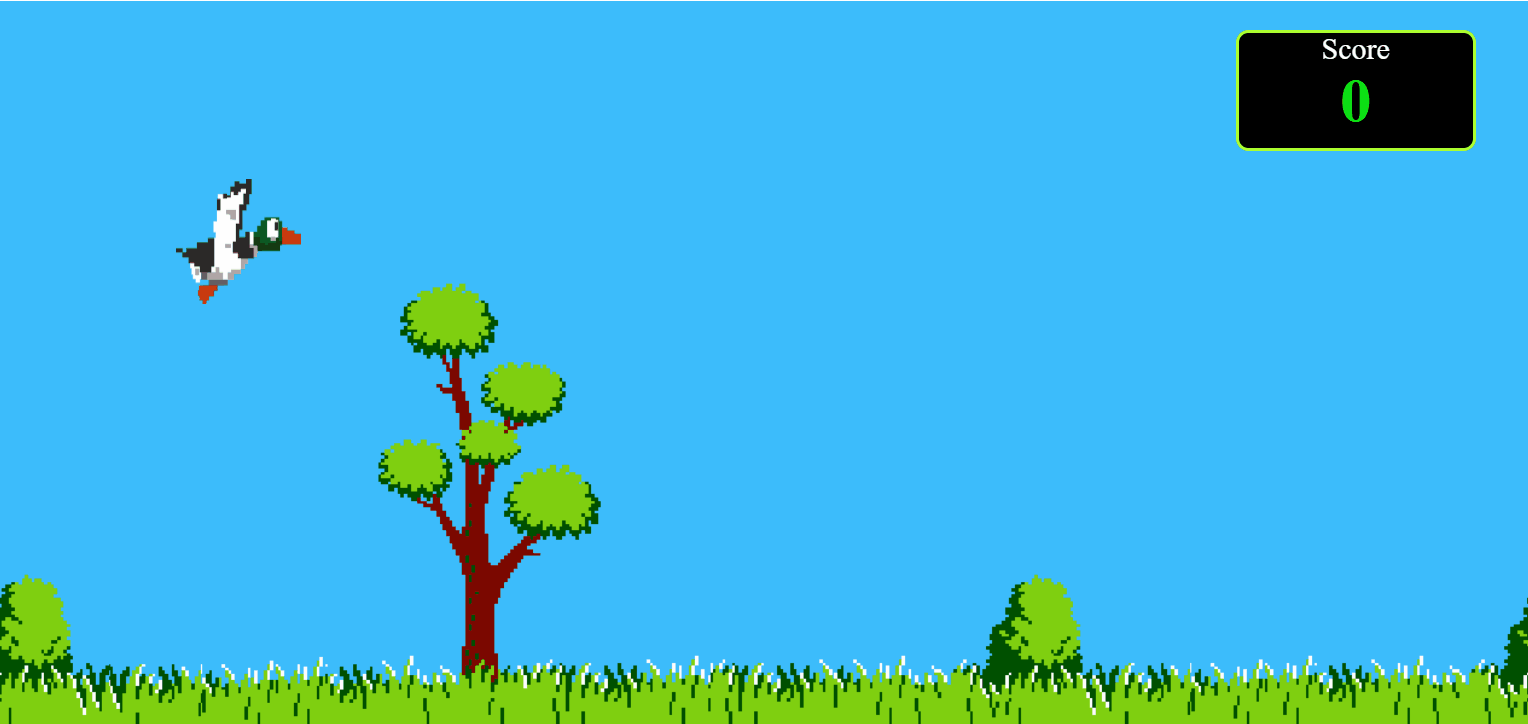
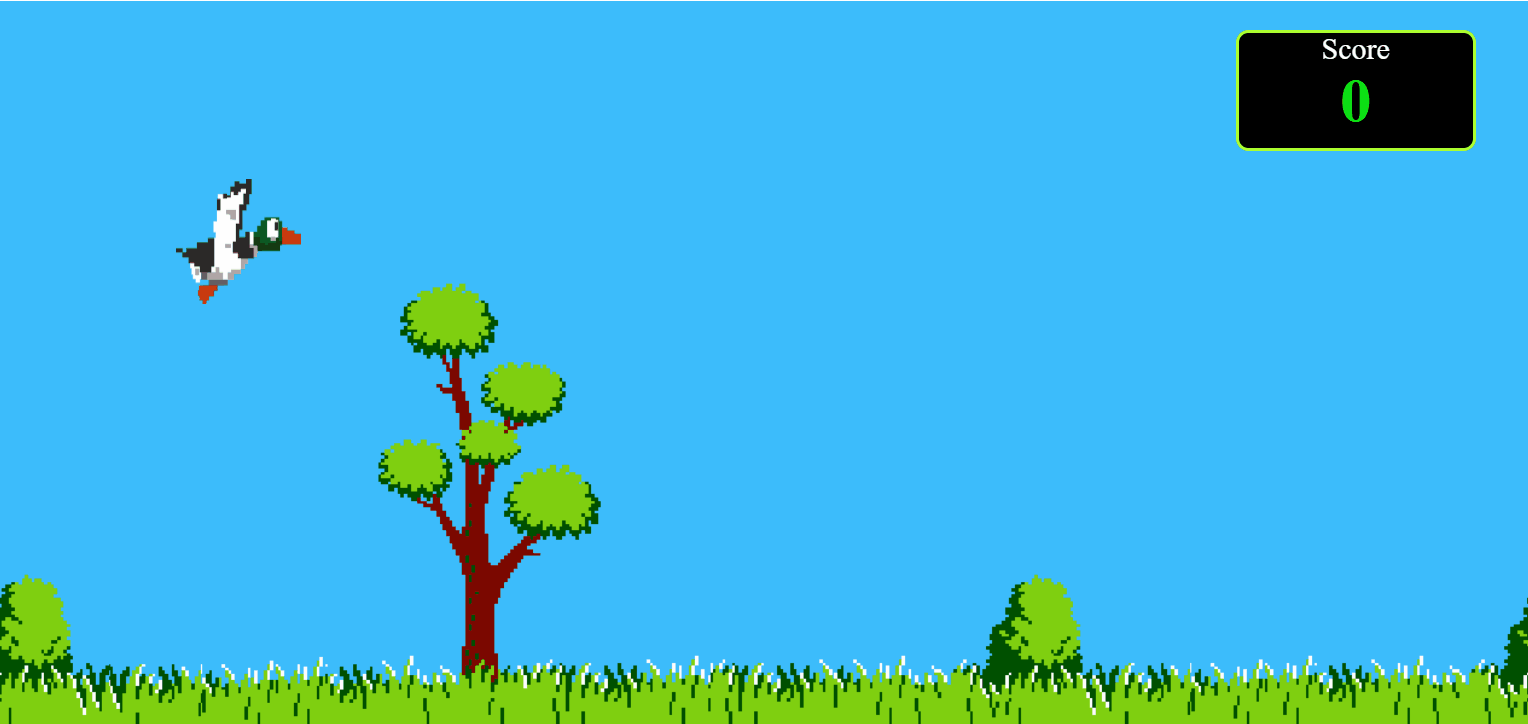
Build out an arcade game with the next requirements
- Use an image as a background and a duck image to click over it.
- When the user clicks on the duck two things should happen:
- Increase the current score by one
- Duck should move to a random position
- Should be build using ES6 specification features
- Use Flexbox for its layout
Pretty simple, isn’t ?. Now what?. It’s a general rule if we are in front of a problem the first thing we need to do is to think about the approach we need to follow and also the recipe, this means to figure out each step and detail we need to complete those requirements. So let’s break this down.
To solve the challenge we will follow the next steps in the given order. Divide and you will conquer!
- Implement the layout using the assets (the background image and the duck) and also the score box.
- What do I need when I click on the duck? You got it, my friend. We will need an event listener. Which will be trigger when we click on it.
- Create a function to increase the current score
- Create a function to move the duck randomly
So, without further ado, let’s dirty our fingers.
1- Layout
Our layout (index.html) will have a div as a container and then both images the background and the duck. Finally, a scoreContainer element with the score text and the score (counter).
<div class="container"> <img src="https://bit.ly/2Q4q14a" /> <img id="duck" src="https://bit.ly/2KQJVKc" alt="duck" /> <div class="scoreContainer"> <div id="score-text">Score</div> <div id="score-counter">0</div> </div> </div>
Styles
/*Make any img element responsive*/ img { max-width: 100%; } /*Set a fixed size for width and height and in an absolute position*/ #duck { margin: 50px; width: 100px; height: 100px; position: absolute; left: 100px; top: 100px; } /*Style for the Score container*/ .scoreContainer { background-color: black; width: 15%; height: 15%; color: #ffffff; top: 5%; right: 5%; border: 2px solid greenyellow; border-radius: 10px; display: flex; position: fixed; flex-direction: column; align-items: center; } #score-text { font-size: 1.5em; } #score-counter { font-size: 3.1em; font-weight: bold; color: #06e515; }
2- JavaScript
2.1 Create the event listener
Now, we are going to create an event listener on our duck image. When a user clicks on the duck image we should fire a function.
//Get the target element const duck = document.querySelector("#duck"); //Add the click event listener duck.addEventListener("click", () => { //Dont forget call the functions here increaseScore(); moveDuck(); });
2.2 Create a function to increase the current score
We just created the event listener, now, we are going to create a function that will help to increase the counter, our score by one.
//Increase score by 1 const increaseScore = () => { //Get the content of the target element. The current value for score const score = document.querySelector("#score-counter").innerHTML; //Get the element to increase the value const scoreHTML = document.querySelector("#score-counter"); //Cast the score value to Number type let count = Number(score); //Set the new score to the target element scoreHTML.innerHTML = count + 1; };
2.3 Create a function to move the duck randomly
It’s time to move the duck. However, since the duck will move in a random way, it’s a good choice to create a helper function to get a random number, which later we are going to use it to set the random position. So, let’s first create that function.
//Get a random number const getRandomNum = (num) => { return Math.floor(Math.random() * Math.floor(num)); }
Wow, we are almost at the end of this incredible practice. Now, we are going to create the function to move the duck. The most important things are: We are using the innerWidth property to get the inner width of the window in pixels and the same for the innerHeight property, we are getting the inner height of the window in pixels. Also, we are using the getRandomNum function to set the pixel numbers for top and left properties to affect the vertical and horizontal position of the duck.
/* Move the duck randomly */ const moveDuck = () => { const w = window.innerWidth; const h = window.innerHeight; duck.style.top = getRandomNum(w) + "px"; duck.style.left = getRandomNum(h) + "px"; };
This all for today my friends. I hope you had followed this simple guide but with a big understanding of DOM manipulation.