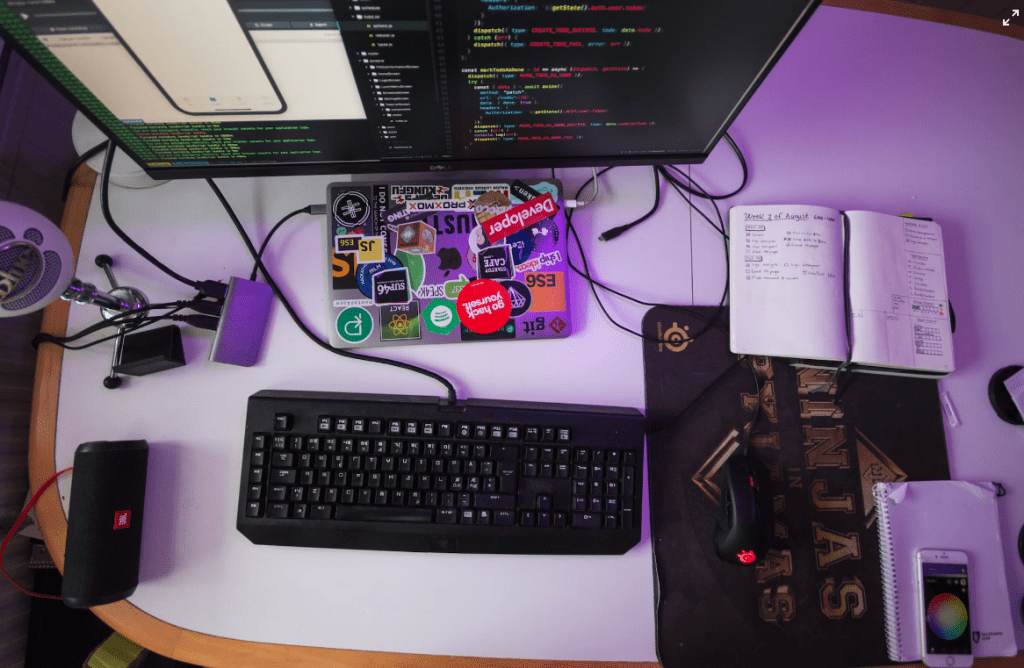
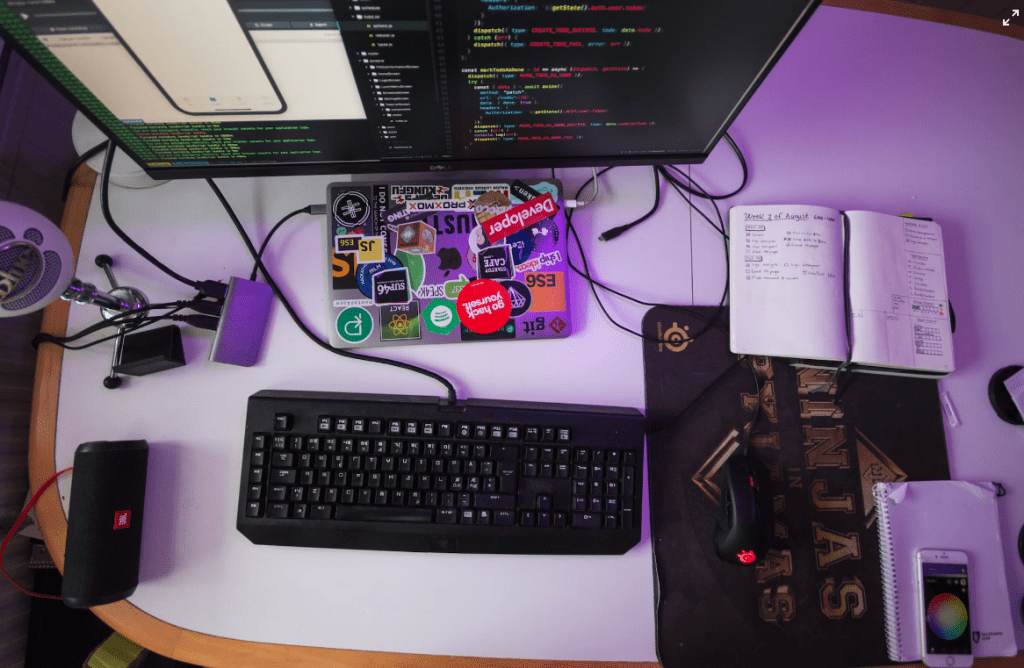
React Native Navigation is a powerful library that allows developers to easily navigate between different screens in a React Native application. In this article, we will go over the basics of using React Native Navigation and explore some of its most useful features.
First, you will need to install the library by running the following command in your terminal:
npm install --save react-navigation
Once the library is installed, you can start using it by importing the necessary components. The main component you will use is the Navigator
component, which will be the parent container for all of your screens.
import { Navigator } from 'react-navigation';
Next, you will need to create a route configuration object, which defines the different screens in your application and the relationships between them. The route configuration object should be an array of objects, where each object represents a screen and has a unique name
and a component
property.
const routeConfiguration = {
Home: {
name: 'Home',
component: HomeScreen
},
Profile: {
name: 'Profile',
component: ProfileScreen
}
};
Once you have defined your route configuration, you can use it in your Navigator
component by passing it as a route
prop.
<Navigator
initialRoute={routeConfiguration.Home}
renderScene={(route, navigator) => {
const Component = route.component;
return <Component navigator={navigator} {...route.passProps} />;
}}
/>
This will render the HomeScreen
as the initial screen when the application is launched. To navigate to other screens, you can use the navigator.push()
method. This method takes an object with a name
property and an optional passProps
property.
navigator.push({
name: 'Profile',
passProps: {
userId: this.state.userId
}
});
This will navigate to the ProfileScreen
and pass the userId
prop to the screen.
In addition to the push()
method, you can also use the pop()
method to go back to the previous screen, or the replace()
method to replace the current screen with a new one.
React Native Navigation also supports tab navigation and drawer navigation out of the box. You can use the TabNavigator
and DrawerNavigator
components to implement these navigation patterns in your application.
Conclusion
Overall, React Native Navigation is a powerful and easy-to-use library that makes it easy to navigate between screens in a React Native application. By following the steps outlined in this article, you should be able to get started with React Native Navigation and start building your own applications.